Integrating Ads into Chatbots
Integrating conversational ads into chatbots offers a unique way to engage users by matching the context of their ongoing conversation with relevant advertising content. Bellow is guide to integrate ChatADy conversational ads using the Python or NPM package.
Overview
Integration requires two types of calls or one in optimized flow. First call is "new chat" which is used to transmit any text sent from user to chatbot and other way around. Second is requesting to retrieve Ad content. Following is a diagram of interactions with optimized (extended new chat) call where new chat call also returns Ad content in single request.
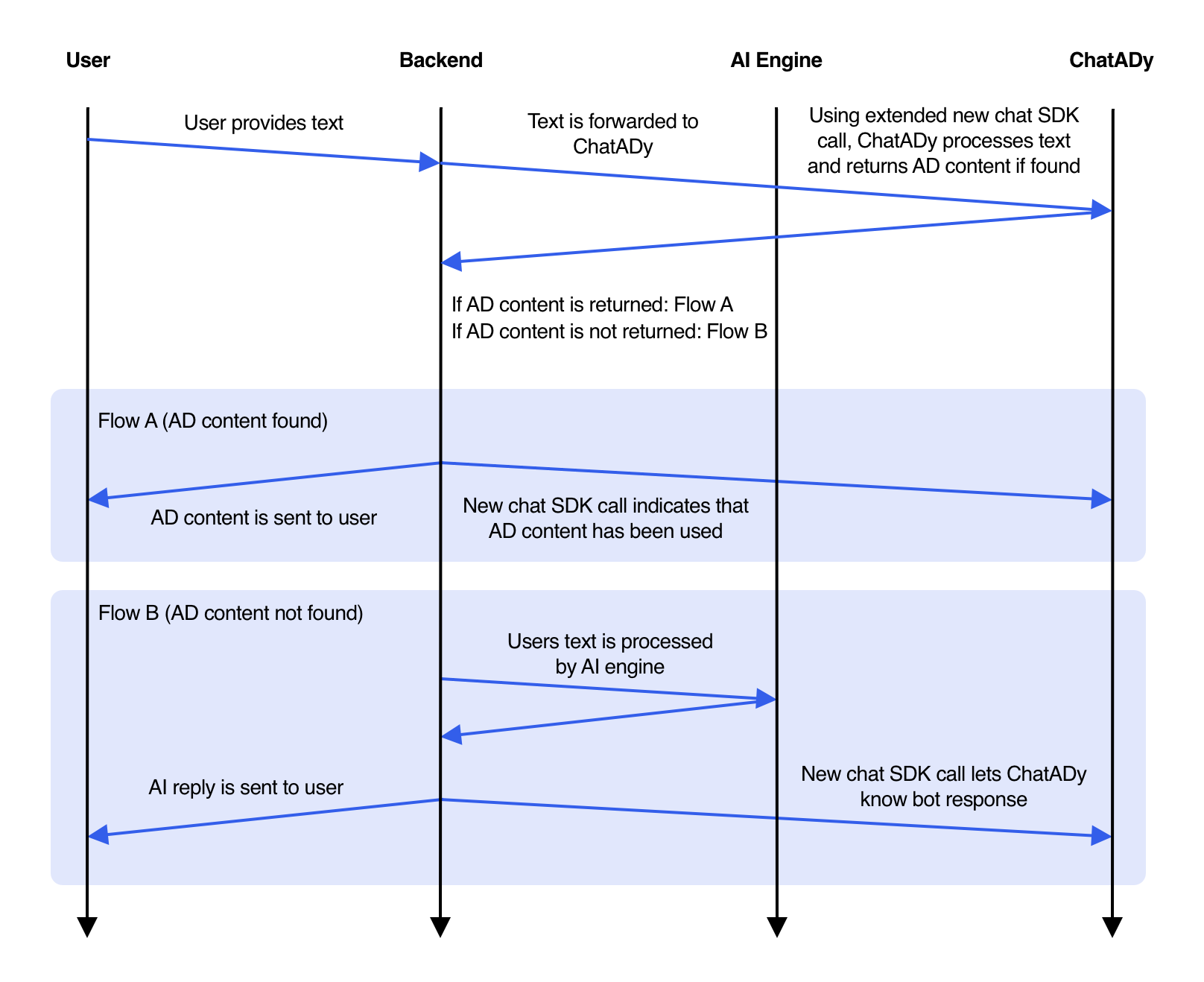
Installation
Install the Python package via pip:
pip install chatady
or install the npm package for Javascript:
npm install chatady-node
Initialize
Initialize the integration with publisher id and api keys obtainable from platform. Execute following for Python integration:
from chatady.chatady import ChatADy
client = ChatADy('your_publisher_id', 'your_api_key')
or following for Javascript:
const ChatADy = require('chatady-node')
const client = new ChatADy('your_publisher_id', 'your_api_key')
Send in chats
Chats betwen user and chatbot are sent in with chat_id that uniquly identifies conversation betwen user and chatbot, users text and True for users text and False for bots text.
With Python:
# send in user text
client.new_chat('chat_id', 'Hello, my sweet Bot!', True)
# extended request that sends in user text with included AD content request in one call
client.new_chat('chat_id', 'Hello, my sweet Bot!', True, { retrieve: True })
# send in bot text (AI text or AD content)
client.new_chat('chat_id', 'Hello, back to you User!', False)
With Javascript:
# send in user text
await client.newChat('chat_id', 'Hello, my sweet Bot!', true)
# extended request that sends in user text with included AD content request in one call
client.new_chat('chat_id', 'Hello, my sweet Bot!', True, { retrieve: true })
# send in bot text (AI text or AD content)
await client.newChat('chat_id', 'Hello, back to you User!', false)
Retrieve Ad content
Get Ad content with Python:
ad_content = client.get_contents('chat_id')
print(ad_content)
# prints with Ad content and earnings in cents if ad is used and found:
# { status: 'success', content: 'Ad content', id: '<ad-id>', earnings: 150 })
# prints if Ad is not found:
# { status: 'success', content: '', id: '', earnings: 0 })
With Javascript:
const response = await client.getContents('chat_id')
console.log(response)
// prints with Ad content and earnings in cents if ad is used and found:
// { status: 'success', content: 'Ad content', id: '<ad-id>', earnings: 150 })
// prints if Ad is not found:
// { status: 'success', content: '', id: '', earnings: 0 })
Wrap-Up and Further Support
Implementing is quite complex and required for proper functioning. Leveraging the chatady libraries in Python or JavaScript can simplify the process. For additional support, guidance, or custom integrations, developers are encouraged to reach out through the library’s GitHub repositories (Python and Javascript) or consult the official ChatADy website.